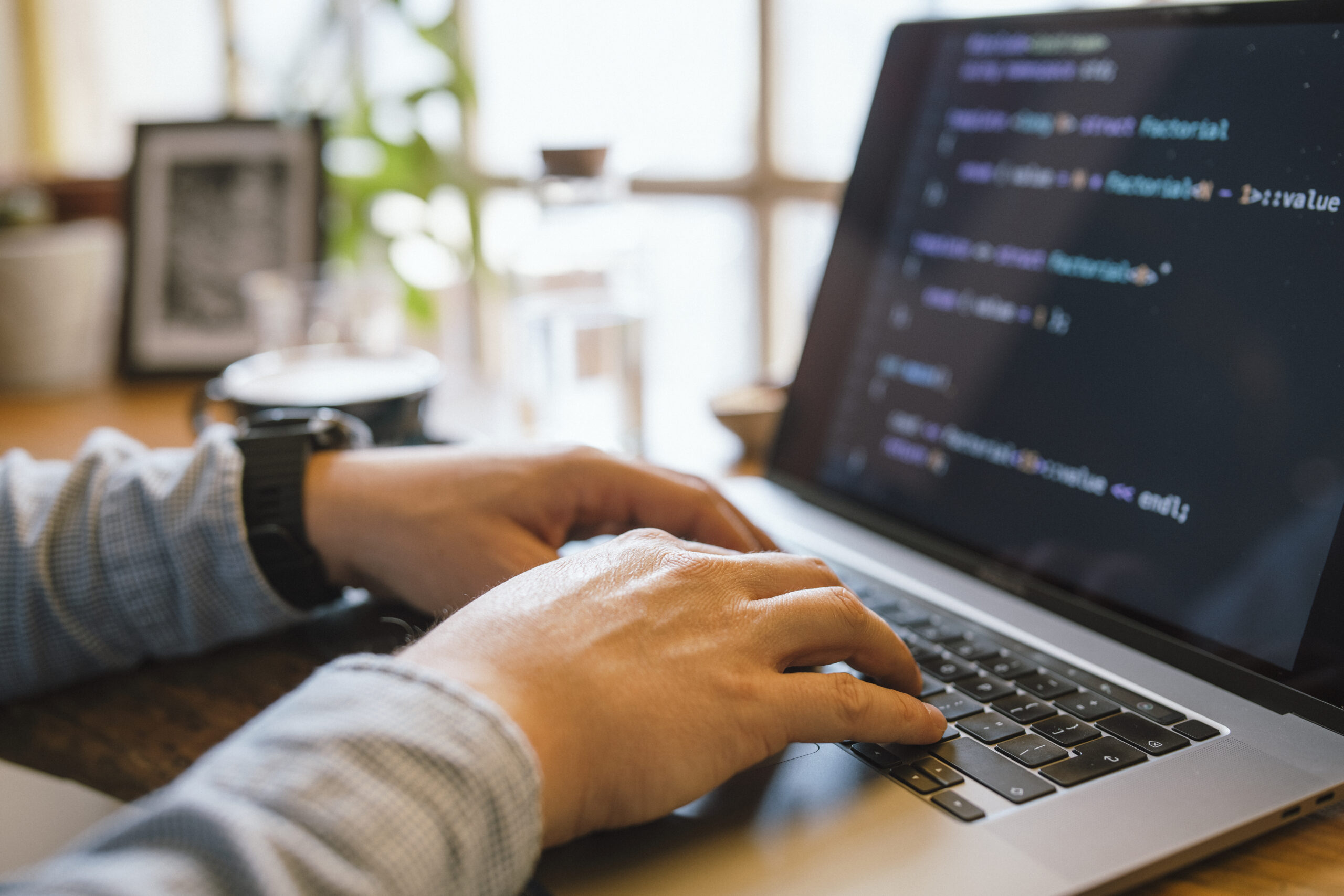
Debugging is Probably the most vital — yet frequently disregarded — techniques inside of a developer’s toolkit. It's not just about fixing broken code; it’s about comprehending how and why items go Mistaken, and Finding out to Believe methodically to solve issues effectively. Regardless of whether you're a newbie or even a seasoned developer, sharpening your debugging expertise can preserve hrs of stress and considerably transform your efficiency. Here i will discuss several strategies that will help builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Resources
Among the quickest means builders can elevate their debugging techniques is by mastering the instruments they use each day. Whilst writing code is one Element of progress, figuring out the way to interact with it effectively through execution is Similarly crucial. Modern enhancement environments appear Outfitted with potent debugging abilities — but several developers only scratch the area of what these resources can perform.
Just take, for instance, an Built-in Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These resources permit you to set breakpoints, inspect the worth of variables at runtime, move as a result of code line by line, and perhaps modify code over the fly. When utilised appropriately, they let you notice specifically how your code behaves during execution, and that is priceless for monitoring down elusive bugs.
Browser developer resources, which include Chrome DevTools, are indispensable for front-end developers. They allow you to inspect the DOM, keep an eye on network requests, perspective actual-time efficiency metrics, and debug JavaScript within the browser. Mastering the console, resources, and network tabs can convert annoying UI issues into manageable tasks.
For backend or program-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Handle around operating processes and memory administration. Learning these equipment could possibly have a steeper Studying curve but pays off when debugging functionality challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, turn into cozy with Model Regulate methods like Git to grasp code heritage, obtain the exact moment bugs had been launched, and isolate problematic variations.
Ultimately, mastering your resources implies heading outside of default options and shortcuts — it’s about producing an personal expertise in your development atmosphere to ensure when challenges crop up, you’re not shed in the dark. The greater you realize your tools, the more time you are able to devote fixing the actual difficulty as opposed to fumbling by means of the method.
Reproduce the trouble
Just about the most crucial — and often overlooked — steps in effective debugging is reproducing the condition. Right before leaping in the code or generating guesses, developers require to produce a dependable natural environment or situation in which the bug reliably appears. Without reproducibility, correcting a bug gets a match of likelihood, typically leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as feasible. Question concerns like: What steps resulted in The difficulty? Which atmosphere was it in — enhancement, staging, or generation? Are there any logs, screenshots, or error messages? The greater depth you've, the a lot easier it gets to isolate the exact ailments below which the bug takes place.
After you’ve gathered ample info, seek to recreate the situation in your local atmosphere. This may imply inputting a similar info, simulating identical user interactions, or mimicking process states. If the issue appears intermittently, take into consideration creating automatic tests that replicate the edge conditions or state transitions included. These tests not merely assistance expose the trouble but will also stop regressions Sooner or later.
Sometimes, The problem can be atmosphere-precise — it might transpire only on certain working programs, browsers, or less than certain configurations. Working with tools like virtual equipment, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating these bugs.
Reproducing the trouble isn’t merely a step — it’s a attitude. It necessitates patience, observation, as well as a methodical technique. But when you finally can continuously recreate the bug, you're presently halfway to repairing it. By using a reproducible state of affairs, you can use your debugging resources much more effectively, check probable fixes safely and securely, and converse extra Evidently with your team or customers. It turns an abstract grievance into a concrete challenge — and that’s exactly where developers prosper.
Browse and Have an understanding of the Mistake Messages
Mistake messages are frequently the most useful clues a developer has when anything goes Improper. As opposed to viewing them as frustrating interruptions, builders really should understand to deal with error messages as immediate communications with the process. They often show you just what exactly transpired, wherever it transpired, and from time to time even why it took place — if you understand how to interpret them.
Start off by looking through the message cautiously and in comprehensive. Quite a few developers, specially when less than time force, glance at the first line and straight away start off creating assumptions. But further while in the error stack or logs may well lie the real root trigger. Don’t just duplicate and paste error messages into search engines like google — read and understand them very first.
Break the mistake down into areas. Could it be a syntax error, a runtime exception, or simply a logic mistake? Will it issue to a selected file and line range? What module or operate triggered it? These questions can manual your investigation and point you towards the responsible code.
It’s also handy to comprehend the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java usually abide by predictable patterns, and Discovering to recognize these can considerably speed up your debugging approach.
Some faults are vague or generic, and in All those circumstances, it’s important to examine the context during which the mistake happened. Check connected log entries, enter values, and up to date modifications while in the codebase.
Don’t ignore compiler or linter warnings either. These usually precede larger concerns and supply hints about prospective bugs.
Finally, error messages will not be your enemies—they’re your guides. Mastering to interpret them correctly turns chaos into clarity, assisting you pinpoint troubles faster, minimize debugging time, and turn into a extra economical and confident developer.
Use Logging Wisely
Logging is Probably the most effective equipment in a very developer’s debugging toolkit. When made use of successfully, it provides genuine-time insights into how an application behaves, assisting you realize what’s taking place under the hood without needing to pause execution or step through the code line by line.
A good logging technique starts with understanding what to log and at what level. Typical logging ranges consist of DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic info throughout development, Facts for typical gatherings (like profitable commence-ups), WARN for potential challenges that don’t crack the appliance, Mistake for true difficulties, and FATAL in the event the process can’t keep on.
Stay away from flooding your logs with excessive or irrelevant details. Far too much logging can obscure critical messages and slow down your procedure. Center on crucial occasions, point out alterations, input/output values, and significant selection details with your code.
Format your log messages Plainly and constantly. Include context, for instance timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting This system. They’re especially precious in production environments wherever stepping via code isn’t doable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about harmony and clarity. Which has a effectively-considered-out logging approach, it is possible to lessen the time it takes to spot difficulties, gain deeper visibility into your apps, and Increase the General maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To proficiently identify and resolve bugs, developers ought to approach the process just like a detective resolving a secret. This state of mind will help stop working complex problems into manageable areas and observe clues logically to uncover the foundation induce.
Begin by collecting evidence. Consider the indicators of the situation: error messages, incorrect output, or overall performance concerns. Similar to a detective surveys a criminal offense scene, accumulate just as much appropriate facts as you could without the need of leaping to conclusions. Use logs, take a look at conditions, and person stories to piece jointly a transparent photo of what’s occurring.
Following, kind hypotheses. Request oneself: What could be creating this actions? Have any changes recently been created to the codebase? Has this concern occurred in advance of underneath related situation? The purpose should be to slender down alternatives and identify opportunity culprits.
Then, take a look at your theories systematically. Endeavor to recreate the situation in a very controlled environment. For those who suspect a certain operate or component, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, request your code questions and Enable the outcome lead you nearer to the truth.
Pay back shut focus to little information. Bugs usually hide from the least envisioned areas—similar to a missing semicolon, an off-by-1 mistake, or perhaps a race ailment. Be comprehensive and affected individual, resisting the urge to patch The problem without thoroughly knowing it. Non permanent fixes may perhaps conceal the actual issue, just for it to resurface later.
And lastly, maintain notes on That which you attempted and figured out. Just as detectives log their investigations, documenting your debugging course of action can conserve time for upcoming problems and assistance Other folks understand your reasoning.
By pondering similar to a detective, developers can sharpen their analytical capabilities, solution issues methodically, and develop into more practical at uncovering hidden troubles in complex devices.
Compose Assessments
Writing assessments is among the most effective strategies to improve your debugging capabilities and In general improvement effectiveness. Assessments don't just aid capture bugs early but also serve as a security net that provides you confidence when building modifications towards your codebase. A well-examined application is much easier to debug mainly because it enables you to pinpoint particularly exactly where and when an issue happens.
Begin with device assessments, which focus on specific capabilities or modules. These tiny, isolated assessments can promptly expose regardless of whether a certain bit of logic is Performing as envisioned. When a test fails, you instantly know in which to search, drastically lowering time expended debugging. Device exams are Specifically beneficial for catching regression bugs—troubles that reappear soon after Formerly becoming fixed.
Up coming, integrate integration exams and finish-to-stop tests into your workflow. These help make certain that a variety of elements of your application perform collectively efficiently. They’re specifically handy for catching bugs that manifest in sophisticated devices with many elements or products and services interacting. If a little something breaks, your assessments can inform you which Section of the pipeline failed and under what conditions.
Crafting assessments also forces you to Believe critically regarding your code. To test a function correctly, you need to be aware of its inputs, anticipated outputs, and edge instances. This degree of knowing Normally potential customers to higher code structure and less bugs.
When debugging a difficulty, composing a failing exam that reproduces the bug might be a powerful starting point. After the test fails persistently, you can give attention to correcting the bug and observe your take a look at pass when The problem is fixed. This strategy ensures that precisely the same bug doesn’t return Sooner or later.
In short, producing checks turns debugging from the aggravating guessing video game into a structured and predictable method—supporting you capture more bugs, more quickly plus much more reliably.
Take Breaks
When debugging a tricky concern, it’s effortless to be immersed in the condition—staring at your display for hrs, striving Option just after solution. But Just about the most underrated debugging equipment is actually stepping absent. Having breaks allows you reset your intellect, reduce aggravation, and often see the issue from a new viewpoint.
When you're also near the code for much too extensive, cognitive exhaustion sets in. You may perhaps start overlooking obvious errors or misreading code that you wrote just several hours before. During this point out, your Mind gets considerably less productive at difficulty-solving. A brief wander, a espresso split, or perhaps switching to a special task for ten–quarter-hour can refresh your emphasis. Several developers report getting the foundation of a difficulty once they've taken time to disconnect, permitting their subconscious get the job done while in the track record.
Breaks also help reduce burnout, In particular for the duration of for a longer time debugging sessions. Sitting down in front of a screen, mentally trapped, is not merely unproductive but also draining. Stepping absent lets you return with renewed Vitality and a clearer way of thinking. You could possibly abruptly notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
In case you’re stuck, a very good guideline is to established a timer—debug actively for 45–60 minutes, then have a 5–ten moment split. Use that point to move all-around, stretch, or do a little something unrelated to code. It could feel counterintuitive, Specially under restricted deadlines, however it essentially results in speedier and more effective debugging In the long term.
In short, getting breaks is not a sign of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your viewpoint, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is really a psychological puzzle, and relaxation is part of solving it.
Study From Every Bug
Every single bug you come upon is more than just A brief setback—It is really an opportunity to expand for a developer. Whether or not it’s a syntax error, a logic flaw, or maybe a deep architectural difficulty, each one can educate you anything precious if you take some time to mirror and review what went wrong.
Begin by asking by yourself a handful of key queries after the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved practices like unit tests, code reviews, or logging? The answers often reveal blind places in your workflow or understanding and assist you to Develop more powerful coding routines shifting forward.
Documenting bugs can also be an excellent habit. Keep a developer journal or maintain a log in which you Take note down bugs you’ve encountered, the way you solved them, and That which you figured out. After a while, you’ll start to see patterns—recurring problems or common mistakes—that you can proactively stay clear of.
In workforce environments, sharing That which you've figured out from a bug along with your peers can be Primarily highly effective. No matter whether it’s through a Slack information, a brief create-up, or A fast know-how-sharing session, aiding Other people steer clear of the similar concern boosts team performance and cultivates a more robust Studying society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as vital elements of your enhancement journey. All things considered, a few of the most effective developers are usually not the ones who write best code, but those that repeatedly discover from their faults.
In the end, check here Just about every bug you repair provides a new layer to the skill set. So future time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, extra capable developer as a consequence of it.
Conclusion
Bettering your debugging competencies requires time, exercise, and tolerance — nevertheless the payoff is big. It will make you a more productive, self-assured, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be better at Everything you do.